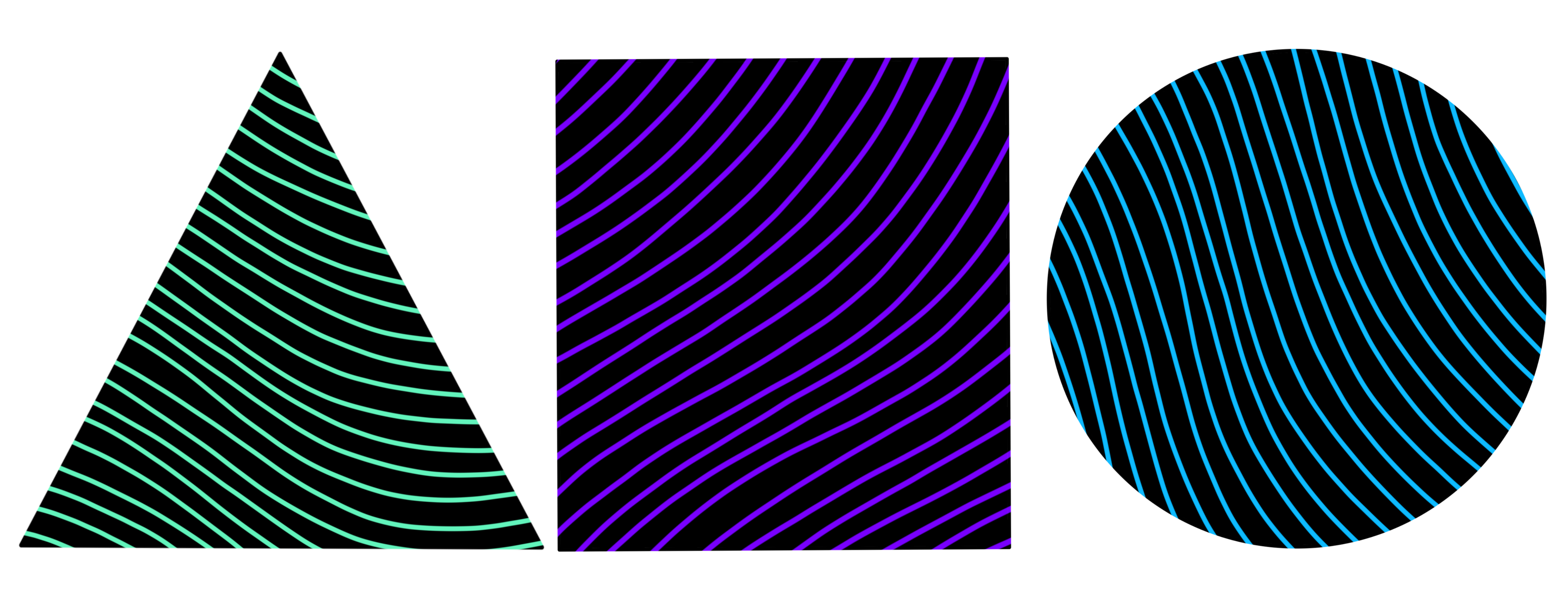
Write JavaScript with Enums
Without any pain or setup
Installation
npm i fun-enums
Usage
const { enums } = require('fun-enums');
// Default initialization
const weekdays = enums()("monday", "tuesday", "wednesday", "thursday", "friday");
weekdays.monday; // 0
weekdays.friday; // 4
Enum API
Initializers
fun-enums
is built around "initializers" which
allow you to change the behavior of how the enum is created. The
default initalizer is number
which sets the first
enum value to 0 and increments each following by 1.
fun-enums
also exposes a
string
initializer which sets the value equal to
the given enum name.
const { enums, string, number } = require('fun-enums');
// Provide other initializers from the package like string
let colors = enums(string)('red', 'green', 'blue')
colors.red; // 'red'
colors = enums(number)('red', 'green', 'blue'); // number does not need to be specified as it is the default behavior
colors.red // 0
You can also provide your own custom initializers by passing in an initialization function to enums.
const { enums } = require('fun-enums');
function capitalize(en, _prevValue) {
return en.charAt(0).toUpperCase() + en.slice(1);
}
const colors = enums(capitalize)('red', 'green', 'blue');
colors.red; // 'Red'
Your initializer will be passed these arguments:
arg | type | description |
---|---|---|
enum | string | the string value passed to the enum to be used as the key |
prevValue | any | the value of the previous enum initialized. Used to increment enum values |
Overrides
In some cases you want a bit more control over the exact values on your enum. You can override the normal behavior of any given initializer by passing in an object rather than an array.
const { enums } = require('fun-enums');
const colors = enums()({ red: '#f44242', green: '#27c65a', blue: '#003bff' });
colors.red; // '#f44242'
This allows you to give values when running a function may not be the best method for defining the enum. You can also "opt-in" to the initializer whenever you want even when overriding by giving a key undefined.
const { enums, string } = require('fun-enums');
const names = enums(string)({ john: 'johnny', sarah: undefined, tim: 'timothy' });
names.sarah; // 'sarah'
names.tim; // 'timothy'
Keys
.keys()
Return a new array with the keys from the enum.
const { enums } = require('fun-enums');
const colors = enums()('red', 'green', 'blue');
colors.keys(); // ['red', 'green', 'blue']
Values
.values()
Return a new array with the values from the enum.
const { enums } = require('fun-enums');
const colors = enums()('red', 'green', 'blue');
colors.values(); // [0, 1, 2]
Entries
.entries()
Return a new array with tuple arrays with the key and value from items in the enum.
const { enums } = require('fun-enums');
const colors = enums()('red', 'green', 'blue');
colors.entries(); // [['red', 0], ['green', 1], ['blue', 2]]
Has
.has()
Returns a boolean if the enum contains the given key.
const { enums } = require('fun-enums');
const colors = enums()('red', 'green', 'blue');
colors.has('red'); // true
colors.has('purple'); // false
Has Value
.hasValue()
Returns a boolean if the enum contains the given value.
const { enums } = require('fun-enums');
const colors = enums()('red', 'green', 'blue');
colors.hasValue(0); // true
colors.hasValue('red'); // false
Get Name
.getName()
Return the string value of the key for a given value.
const { enums } = require('fun-enums');
const colors = enums()('red', 'green', 'blue');
colors.getName(0); // 'red'
colors.getName(99); // undefined
if (hello) {
then('we party', () => {
return 'all night';
})
}